React 项目中实现自定义右键菜单
2022-02-10 14:55:42
React 项目中实现自定义右键菜单,代码如下:
```
import React, {Component} from 'react';
class Example extends Component {
constructor(props) {
super(props);
this.state = {
visible: false,
};
}
componentDidMount() {
// 添加右键点击、点击事件监听
document.addEventListener('contextmenu', this.handleContextMenu)
document.addEventListener('click', this.handleClick)
}
componentWillUnmount() {
// 移除事件监听
document.removeEventListener('contextmenu', this.handleContextMenu)
document.removeEventListener('click', this.handleClick)
}
// 右键菜单事件
handleContextMenu = (e) => {
e.preventDefault()
this.setState({visible: true})
// 获取当前鼠标位置
const clickX = e.clientX
const clickY = e.clientY
// 修改菜单的位置到当前鼠标位置
this.menuDom.style.left = `${clickX}px`
this.menuDom.style.top = `${clickY}px`
};
// 鼠标单击事件,当鼠标在任何地方单击时,设置菜单不显示
handleClick = () => {
const {visible} = this.state
if (visible) {
this.setState({visible: false})
}
}
render() {
return (
<>
{this.state.visible ?
<div ref={(ref) => {
this.menuDom = ref
}} style={{
position: 'absolute',
zIndex: 1000,
backgroundColor: '#656267',
color: '#fff',
borderRadius: 5,
padding: 10,
}}>
<div>关闭</div>
<div>关闭其他</div>
<div>关闭右侧</div>
<div>关闭全部</div>
</div> : ''}
</>
)
}
}
export default Example;
```
效果如图:
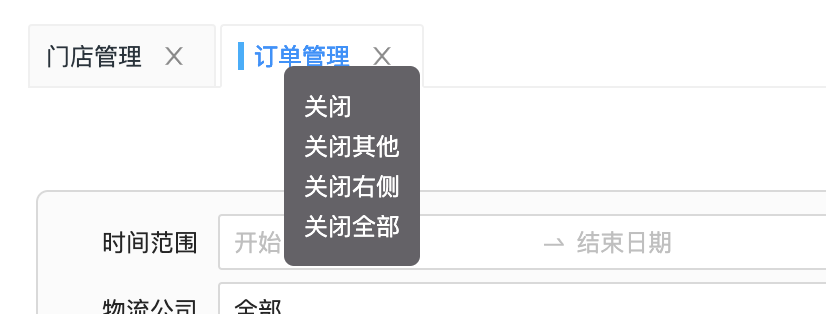